动态 (36)
Rainbow
2023年06月20日
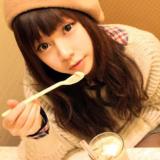
Introduction
Input and output operations involving files are fundamental in many C++ applications. Whether you need to read data from a file, write data to a file, or manipulate files in various ways, the C++ File Input and Output (I/O) capabilities provide a robust and flexible solution. In this article, we will explore the basics of file I/O in C++ and discuss techniques to efficiently handle file operations.1. Opening and Closing Files
Before performing any file I/O operations, you need to open the file using the `std::ifstream` (for reading) or `std::ofstream` (for writing) classes. To open a file, you specify its name and optionally the mode (e.g., `std::ios::in` for input or `std::ios::out` for output). For example: ```cpp #include2. Reading from Files
To read data from a file, you can use various techniques depending on the format and structure of the data. The most common approach involves using the extraction operator (`>>`) to extract data from the file into variables of appropriate types. For example: ```cpp int number; inputFile >> number; std::string text; inputFile >> text; ``` Additionally, you can use `std::getline()` to read an entire line of text from a file, which is useful for reading text files line by line.3. Writing to Files
To write data to a file, you can use the insertion operator (`<<`) to insert data into the output stream. For example: ```cpp int number = 42; outputFile << number << std::endl; std::string text = "Hello, world!"; outputFile << text << std::endl; ``` It's important to note that the `std::endl` manipulator is used to insert a newline character and flush the stream buffer.4. Error Handling
When performing file I/O operations, it's crucial to handle potential errors. You can check if a file is successfully opened by checking the stream object's `good()` member function. Additionally, you can use the `fail()` member function to check if an error occurred during a previous operation. It's advisable to validate the success of file operations and handle any errors appropriately to ensure robust and reliable code.5. Binary File I/O
In addition to text-based file I/O, C++ also supports binary file I/O. Binary files allow you to write and read data in its raw form, preserving the exact binary representation of the data. This is useful when working with complex data structures or when precise control over the data is required. Binary file I/O involves using the `std::ifstream` and `std::ofstream` classes with the `std::ios::binary` flag. ```cpp std::ofstream binaryFile("data.bin", std::ios::binary); binaryFile.write(reinterpret_castConclusion
File I/O is a fundamental part of many C++ applications, and mastering the techniques for efficient file handling is essential. By using the `std::ifstream` and `std::ofstream` classes, C++ provides a powerful and flexible framework for reading from and writing to files. Understanding the basics of file I/O operations, error handling, and binary file I/O allows you to effectively manipulate and process file data in your C++ programs.
文章
相关用户